Unity is a renowned platform for game development, offering a plethora of tools and features to craft striking and immersive gaming experiences. To fully utilize the capabilities of Unity, adherence to coding protocols and best practices is essential. These guidelines not only enhance the readability of code but also optimize performance and maintainability, as well as foster improved collaboration within development teams.
The practice of utilizing sound naming conventions within Unity can significantly enhance both the readability and maintainability of your code.
Here are some directives for the naming of various elements within Unity projects:
Game Elements:
- Select informative names that clearly elucidate the element’s function or purpose.
- Steer clear from generic or ambiguous names such as “Object1” or “Cube,” that fail to provide adequate context.
- Ensure the use of a consistent naming approach, such as PascalCase or camelCase.
Example: “PlayerCharacter” or “enemySpawner”.
Data Elements:
- Choose names that accurately depict the data the variable symbolizes.
- Avoid using single-letter or abbreviated names unless they are universally understood and commonly employed.
- Begin the variable name with a lowercase letter or employ camelCase.
Example: “score” or “playerHealth.”
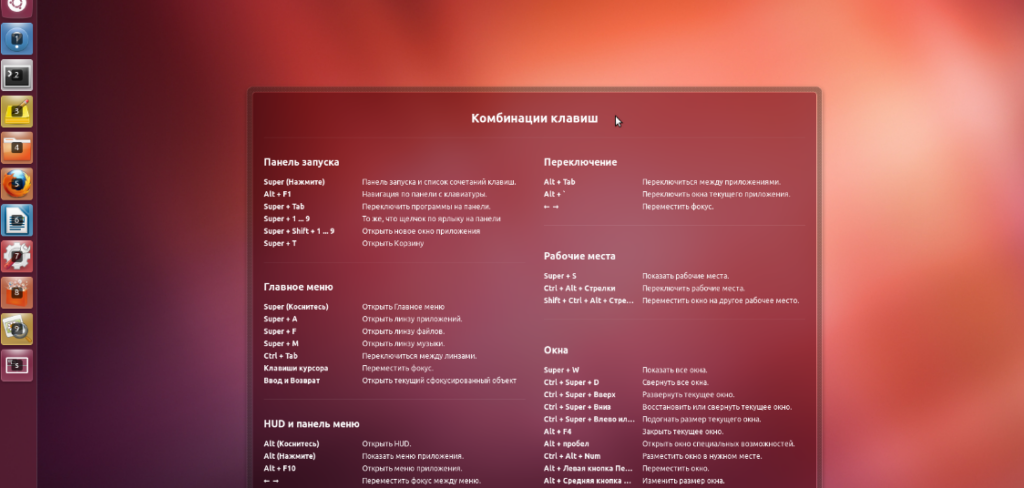
Functionalities and Methods:
- Employ action words or phrases to depict the function performed.
- Use camelCase or PascalCase for function names.
- Select names that are succinct but still describe the function’s purpose.
Example: “MovePlayer()” or “CalculateDamage().”
Classifications and Scripts:
- Utilize nouns or phrases that accurately echo the purpose or functionality of the class.
- Employ PascalCase for class names.
- Use namespaces to arrange classes and avoid naming conflicts.
Example: “PlayerController” or “GameManager.”
Event Triggers and Delegates:
- Employ descriptive names that communicate the purpose of the event or delegate.
- Consider using the “On” or “Event” prefix for clarity in event names.
- Use PascalCase for event and delegate names.
Example: “OnPlayerDeath” or “DamageEvent.”
Enumerations and Constants:
- Use capital letters and underscores for separating words in enumeration and constant names.
- Choose names that accurately represent the values they encompass or define.
- Use descriptive names to enhance code readability.
Example: “DifficultyLevel” or “MAX_HEALTH.”
Strict adherence to these naming conventions can enhance the clarity of your code, making it easier for you and other developers to navigate and maintain your Unity projects. Consistency in naming conventions throughout your project is the key to optimal code readability.
Nine Essential Coding Guidelines & Fundamental Best Practices
Adopting appropriate naming conventions enhances the readability and maintainability of your code. This section discusses naming guidelines for game elements, data elements, functionalities, classifications, and namespaces in Unity projects.
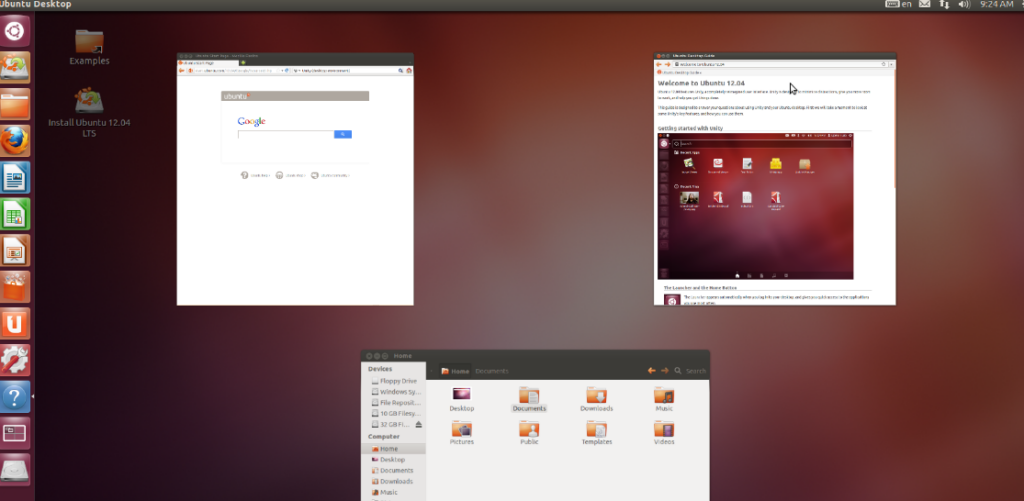
Organizational Structure and File Arrangement
Efficient organization and structuring of Unity project files and folders are vital for effective project management, collaboration, and easy navigation. A well-structured file system allows for easier asset, script, and project element management. This section highlights best practices for the organization and structuring of Unity project files and folders.
Asset Organization:
- Categorize assets based on their type and purpose. Create separate folders for scripts, scenes, prefabs, textures, models, audio, etc.
- Use subfolders within each asset category to further classify the assets. For instance, within the Scripts folder, you can create subfolders for Player, UI, Managers, etc.
- Refrain from overloading the root directory with numerous assets. Maintain a clean directory by organizing assets into relevant folders.
- Consider using the Unity “Packages” folder for managing external packages and plug-ins.
By adhering to these file organization and structure guidelines, you can enhance project management, collaboration, and the overall development workflow within the Unity framework. This approach can lead to more streamlined and manageable projects.
Enhancing Code Readability and Documentation
Code readability and documentation are paramount aspects of software development, Unity-based projects included. Crafting clean and easy-to-understand code enhances teamwork, simplifies maintenance, and elevates the overall quality of the code base. Moreover, well-annotated code assists developers in comprehending the purpose, functionality, and application of various code elements. In this section, we explore best practices for code readability and documentation within the Unity environment.
Maintaining Consistent Formatting and Indentation:
- Establish and maintain a consistent and standardized format throughout your code base.
- Enhance readability by consistently indenting code blocks.
- Consider utilizing tools or code formatters to automatically enforce formatting rules.
Choosing Descriptive Variable and Method Names:
- Select meaningful names that accurately convey the purpose and functionality of variables and methods.
- Steer clear of one-letter variable names or abbreviated names that may not be universally understood.
- Choose names that express intent and clarify the code.
Effective Commenting:
- Use comments to elucidate complex algorithms, business rules, or unintuitive sections of code.
- Highlight important or critical portions of the code with comments.
- Avoid over-commenting or unnecessary comments that clutter the code and provide redundant information.
- Update or eliminate comments when the code changes to ensure they remain accurate and relevant.
Using Documentation Comments:
- Leverage the XML documentation comments in Unity to provide documentation for classes, methods, and variables.
- Thoroughly document public APIs, including input parameters, return values, and usage instructions.
- Describe the purpose, behavior, and potential side effects of methods and classes.
Ensuring Modularity and Code Structure:
- Break down intricate logic into smaller, more manageable functions or methods.
- Use classes and object-oriented principles to encapsulate related functions.
- Adhere to the principle of single responsibility to separate problems and responsibilities.
- Avoid lengthy and confusing methods or classes.
Maintaining Consistent Coding Style:
- Develop and adhere to a coding style guide that covers naming conventions, formatting, and other coding practices.
- Ensure that all team members adopt a consistent coding style to maintain consistency across the project.
Memory Management and Performance Optimization
Efficient memory usage and performance optimization are essential in Unity project development. These elements contribute to a seamless and responsive user experience. In this section, we explore best practices for memory management and performance optimization in the Unity environment.
Implementing Object Pooling:
- Use object pooling to reuse and recycle game objects instead of constantly creating and destroying them.
- Object pooling reduces the overhead of memory allocation and garbage collection, resulting in improved performance.
Avoiding Excessive Instantiation:
- Limit the creation of unnecessary objects, especially during gameplay or runtime.
- Create objects only when necessary and reuse existing objects whenever possible.
Choosing the Right Data Structures:
- Optimize memory usage and performance by selecting the right data structures for different scenarios.
- Use arrays or lists if the number of elements is fixed or changes dynamically, respectively.
- Utilize dictionaries or hash maps for quick data search or retrieval.
Optimizing Memory for Assets
Streamlining Rendering and Draw Calls:
- By grouping several game objects or meshes into a single batch, you can reduce the number of draw calls.
- Optimize rendering performance by utilizing Unity’s static or dynamic batching capabilities.
- Cut down on redrawing by avoiding the rendering of unwanted pixels or transparent objects.
Implementing Levels of Detail (LOD):
- Use LOD systems for 3D models to dynamically tweak the level of detail based on camera distance.
- By reducing the number of polygons and texture resolution for far-off objects, LOD systems can boost performance.
Optimizing Your Code:
- Employ the Unity profiler to profile your code and identify performance hotspots.
- Streamline critical sections of your code by reducing unnecessary calculations, loop iterations, or function calls.
- Opt for algorithms and data structures with optimal temporal complexity for resource-demanding operations.
Using Caching and Memoization Techniques:
- Store frequently accessed data or expensive calculations to avoid repeated computations.
- Leverage memoization methods to store and reuse function results for identical input parameters.
Mobile-Specific Optimization
Profiling and Testing:
- Regularly profile and test your game to spot and rectify performance issues.
- Utilize the Unity profiler or external profiling tools to gauge and analyze performance metrics.
- Ensure a smooth user experience across devices by testing your game on target platforms.
Remember, performance optimization is an iterative process. Continually monitor and optimize your codebase as the project evolves and new performance issues crop up. Striking a balance between optimization and maintainable, readable code is crucial for the project’s long-term success.
Mastering Input Handling and Event Management
Effective input handling and event management are critical to successful game development in Unity, as they can greatly enhance gameplay and interactivity. In this section, we delve into best practices for managing inputs and events within Unity.
Leveraging the Unity Input System:
- Make use of the Unity Input System package for a robust, flexible method of handling user input.
- This system offers improved performance, support for multiple input devices, and a more uniform API.
Input Actions and Bindings:
- Input actions, representing logical game actions such as Jump or Attack, and bindings, defining how these actions are triggered (e.g., a specific key, button, or touch gesture), should be centrally defined.
- Leverage the Input Action Asset of the input system to manage and configure input actions and bindings.
Input Processing Code:
- Keep input processing code encapsulated in separate classes or scripts to maintain its organization.
- Avoid integrating input processing logic directly into MonoBehaviour scripts managing other game functions.
- Adopt a modular and extendable approach to deal with different types of input, such as keyboard, mouse, touch, and gamepad.
Event Delegation and the Observer Pattern:
- Implement an event delegation or observer pattern to decouple event senders from recipients.
- Allow multiple objects to listen and react to events without direct communication or dependencies.
- Convey relevant data or contextual information along with events using event parameters.
Handling User Interface Events:
- Utilize the Unity UI system (e.g., buttons, switches, sliders) to manage user interaction with UI elements.
- To respond to button presses, slider value changes, or other UI events, attach event listeners to UI elements.
- Consider using Unity’s EventTrigger or third-party libraries for managing more complex UI interactions.
Managing Game States:
- Use a state machine or similar architectural pattern to manage the game’s states and transitions.
- Trigger events or state changes based on specific game conditions, such as level completion or player actions.
- Ensure that event listeners are properly cleared and removed when transitioning between game states.
Input Validation and Error Handling:
- Validate and sanitize user input to prevent invalid or unexpected data from influencing the game.
- Implement appropriate error handling and provide meaningful feedback to the user in the event of input errors.
Performance Considerations:
- Avoid continuous polling of input state in update cycles. Use events or callbacks to respond to input changes.
- Optimize event dispatching and event listener logging to minimize performance overhead.
- Profile and analyze the performance of input processing and event management to identify potential bottlenecks.
Testing and Debugging:
- Thoroughly test input processing and event management to ensure correct functionality and responsiveness.
- Utilize Play Mode tests in Unity or automated testing mechanisms to confirm input and event behavior.
- Implement logging and debugging tools to track and troubleshoot input-related issues.
Implementing these best practices for input processing and event management can lead to the creation of engaging and dynamic games in Unity. The effective orchestration of user input and events can result in more fluid gameplay, as well as allow for superior control and tailoring of game mechanics.
Script Communication and Structural Modularity
Structural modularity and script communication are vital for structuring and maintaining Unity projects. Ensuring efficient communication among scripts and keeping modular code can enhance code reuse, debugging ease, and scalability. Here, we explore best practices for script interaction and modularity in Unity.
Assigning Defined Responsibilities to Scripts:
- To enhance modularity and maintainability, design scripts with a single responsibility.
- Each script should focus on a distinct functionality aspect, such as player control, enemy behavior, or UI management.
- Avoid constructing bulky scripts that handle many unrelated functions.
Centralized Managers and Controllers:
- Establish centralized manager or controller scripts to coordinate and manage game systems or specific functionalities.
- Managers can perform tasks like input management, scene transitions, game state management, or sound management.
- Controllers can manage higher-level logic and coordinate interactions between different systems or scenarios.
Referencing Scenarios:
- Utilize scenario references to create communication between scenarios.
- Rather than relying on GameObject.Find or GetComponent calls, directly assign script references in the inspector or in code.
- This method reduces the overhead of finding objects or components at runtime and enhances performance.
Messaging Systems:
- Design messaging systems or event buses to facilitate communication among scripts.
- These systems act as middlemen, receiving and distributing messages or events between scenarios.
- Messages can be used to trigger actions, update states, or transfer data between scenarios.
Interfaces and Abstraction:
- Leverage interfaces and abstraction to define common contracts between scenarios.
- Interfaces establish a contract that outlines the methods or properties that implementing scripts must provide.
- Abstraction enables you to create base classes or scripts with shared functionality that can be inherited or extended by other scripts.
Loose Coupling and Dependency Injection:
- Aim for loose coupling among scripts to reduce dependencies and increase flexibility.
- Employ dependency injection patterns to supply necessary dependencies to scripts instead of hardcoding references.
- Dependency injection frameworks or container libraries can simplify this process.
Modularity and Code Organization:
- Arrange your scripts into logical folders or namespaces based on their functionality or purpose.
- Employ naming conventions and folder structures that make script files easy to locate and understand.
- Group related scripts together to enhance code readability and maintainability.
Documentation:
- Document communication protocols and dependencies between scripts.
- Offer clear instructions for using the scripts and document any requirements or limitations.
- Use comments and summaries to explain the scripts’, methods’, and variables’ purpose and functionality.
By adhering to these best practices for interacting scripts and achieving modularity in Unity, you can produce well-structured, reusable, and maintainable code. This method facilitates collaboration, debugging, and future improvements in your Unity projects.
Asset Management and Optimization
Asset management and optimization are crucial to developing efficient and productive Unity projects. Assets, such as textures, models, audio files, and animations, influence your game’s overall size and performance.
Asset Import Settings:
Customize import settings for each asset type to optimize their utilization.
Unity offers various import settings for textures, models, audio files, etc., allowing you to manage compression, quality, MIP mapping, and other parameters.
Adjust the settings to meet your project’s specific requirements, striking a balance between quality and performance.
Texture Compression:
- Employ texture compression techniques to decrease memory usage and enhance performance.
- Use compressed texture formats (e.g., DXT, ETC, ASTC) for different platforms to achieve a balance between quality and performance.
- Modify the size and resolution of textures according to the requirements of the target platform.
Texture Atlas Creation:
- Combine several smaller textures into a larger texture atlas.
- A texture atlas reduces the number of drawing and switching calls, enhancing render performance.
- Utilize tools such as Unity’s Sprite Packer or third-party atlas creation tools to automate the process.
Asset Bundles:
Leverage asset bundles for dynamic asset management and loading.
Partition assets into bundles based on usage patterns or levels to diminish initial load times.
Load asset bundles asynchronously to prevent obstructing the main flow and to ensure a seamless user experience.
Texture and Material Sharing:
- Aim to share textures and materials across multiple objects or components whenever feasible.
- Reuse materials with identical shaders and settings to minimize GPU memory usage.
- Utilize texture atlases or texture arrays for interchangeable textures among objects.
By adhering to these best practices for asset management and optimization, you can craft Unity projects that excel in memory usage, load time, and overall performance. Effective asset management guarantees that your game will run fluidly across various platforms and devices, all while maintaining a superior level of visual quality.
Conclusion
In essence, abiding by coding guidelines and best practices is crucial to crafting efficient and user-friendly Unity code. Compliance with these guidelines will enhance collaboration, performance, and the overall development experience, leading to top-tier games.
Implement these best practices and unlock Unity’s full potential to create exceptional games for players globally.