Understanding EventTrigger Components in Unity
EventTrigger is a powerful component in Unity that allows developers to intercept and respond to various events triggered by user interactions. In this article, we will delve into the fundamentals of EventTrigger components, exploring their capabilities, usage, and the different types of events they can handle. By the end, you will have a solid understanding of how EventTrigger works and how you can leverage its functionality to create immersive and interactive experiences in your Unity projects.
The EventTrigger component in Unity serves as a receiver for events from the EventSystem, enabling the triggering of registered functions based on specific events. With EventTrigger, you have the flexibility to assign multiple events and determine their execution order. This powerful functionality allows you to precisely specify the functions to be called for each event, facilitating intricate event-driven interactions within your project.
There are various event types in Unity that can be intercepted using EventTrigger:
- PointerEnter: Called when the mouse pointer is over the Game Object.
- PointerDown: Triggered when a pointer button is pressed.
- PointerUp: Triggered when a pointer button is released.
- PointerExit: Called when the mouse pointer exits the Game Object.
- PointerClick: Triggered when a pointer button is clicked.
- Drag: Invoked when a drag is being performed.
- Drop: Called when an object is dropped during a drag-and-drop operation.
- Scroll: Triggered when the mouse scroll wheel is scrolled.
- BeginDrag: Called at the start of a drag operation.
- EndDrag: Triggered at the end of a drag operation.
- Initialized Potential Drag: Invoked when a potential drag is initialized.
- Deselect: Called when the Game Object is deselected.
- Select: Triggered when the Game Object is selected.
- Submit: Invoked when a form is submitted.
1.1 PointerEnter Event
The PointerEnter event is triggered when the mouse pointer hovers over a Game Object. It serves as a powerful trigger in Unity, activating when the mouse pointer gracefully glides over a Game Object. This event opens up a world of possibilities, allowing developers to seamlessly introduce dynamic responses and interactions based on the user’s hovering behavior. Whether it’s highlighting interactive elements, providing tooltips, or initiating contextual actions, harnessing the PointerEnter event empowers developers to create immersive and intuitive user experiences that captivate and engage players. Embrace the potential of the PointerEnter event and bring your Unity projects to life with enhanced interactivity and user engagement.
Demonstration:
- Add an Image component to the scene. Right-click in the property window, select UI, and choose Image.
- Select the Image and click on “Add Component” in the Inspector window.
- Search for “Event Trigger” and select it from the options in the property window.
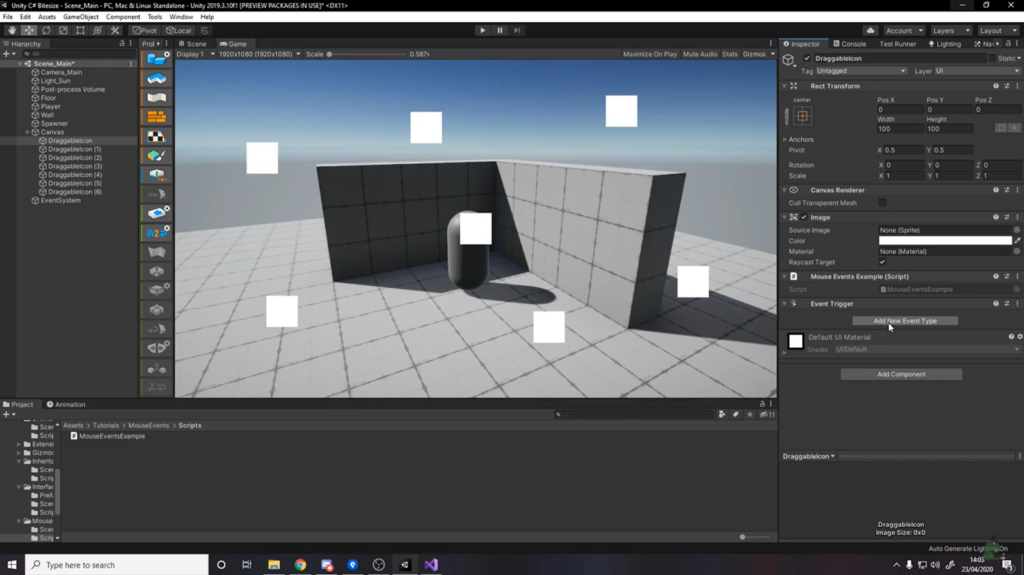
- Click on “Add New Event Type” in the Event Trigger component.
- Choose the PointerEnter event from the dropdown menu.
- Now, the setup should resemble the provided image.
- Create a script and attach it to a game object. The setup should resemble the provided image.
- Copy the script below into the “TextInput” function.
- Select the Image and click on the “+” icon to add an event.
- Drag the “Text Input script” from the Hierarchy window to the PointerEnter event.
- Select onPointerClickEventTrigger() function from PointerEnter Event.
- Now Run the project. When your pointer is above image the function is called.
1.2 PointerDown Event
The PointerDown event is triggered when the mouse button is pressed down on the Game Object.
Demonstration:
- Add an Image component to the scene. Right-click in the property window, select UI, and choose Image.
- Select the Image and click on “Add Component” in the Inspector window.
- Search for “Event Trigger” and select it from the options in the property window.
- Click on “Add New Event Type” in the Event Trigger component.
- Choose the PointerDown event from the dropdown menu.
- Now, the setup should resemble the provided image.
- Create a script and attach it to a game object. The setup should resemble the provided image.
- Copy the script below into the “TextInput” function.
using System.Collections; | |
using System.Collections.Generic; | |
using UnityEngine; | |
using UnityEngine.UI; | |
public class textInput : MonoBehaviour | |
{ | |
//this function is called When a dropdown item is selected. | |
public void onPointerEnterEventTrigger() | |
{ | |
print(“Pointer Enter Event Call……”); | |
} | |
} |
- Select the Image and click on the “+” icon to add an event.
- Drag the “Text Input script” from the Hierarchy window to the PointerDown event.
- Select the onPointerDownEventTrigger() function from the Event.
- Run the project. The function will be called when the mouse button is pressed down on the Image.
1.3 PointerUp Event
The PointerUp event is triggered when the mouse button is released from the Game Object.
Demonstration:
- Add an Image component to the scene. Right-click in the property window, select UI, and choose Image.
- Select the Image and click on “Add Component” in the Inspector window.
- Search for “Event Trigger” and select it from the options in the property window.
- Click on “Add New Event Type” in the Event Trigger component.
- Choose the PointerUp event from the dropdown menu.
- Now, the setup should resemble the provided image.
- Create a script and attach it to a game object. The setup should resemble the provided image.
- Copy the script below into the “TextInput” function.
using System.Collections; | |
using System.Collections.Generic; | |
using UnityEngine; | |
using UnityEngine.UI; | |
public class textInput : MonoBehaviour | |
{ | |
public void onPointerDownEventTrigger() | |
{ | |
print(“Pointer Drag Event Call……”); | |
} | |
} |
- Select the Image and click on the “+” icon to add an event.
- Drag the “Text Input script” from the Hierarchy window to the PointerUp event.
- Select the onPointerUpEventTrigger() function from the Event.
- Run the project. The function will be called when the mouse button is released from the Image.
1.4 PointerExit Event
The PointerExit event is triggered when the mouse pointer exits from the Game Object.
Demonstration:
- Select the Image in the Unity editor.
- Click on “Add Component” in the Inspector window.
- Search for “Event Trigger” and select it from the options in the property window.
- Click on “Add New Event Type” in the Event Trigger component.
- Choose the PointerExit event from the dropdown menu.
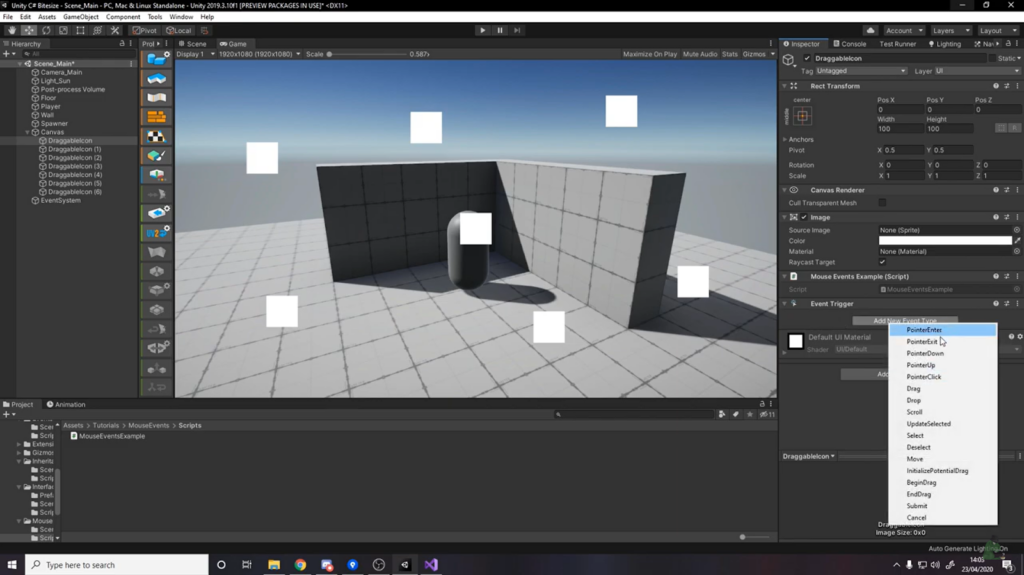
- Now, the setup should resemble the provided image.
- Create a script and attach it to a game object. The setup should resemble the provided image.
- Copy the script below into the “TextInput” function
using System.Collections; | |
using System.Collections.Generic; | |
using UnityEngine; | |
using UnityEngine.UI; | |
public class textInput : MonoBehaviour | |
{ | |
public void onPointerUpEventTrigger() | |
{ | |
print(“Pointer Enter Event Call……”); | |
} | |
} |
- Select the Image and click on the “+” icon to add an event.
- Drag the “Text Input script” from the Hierarchy window to the PointerExit event.
- Select the onPointerExitEventTrigger() function from the Event.
- Run the project. The function will be called when the mouse pointer exits from the Image.
1.5 PointerClick Event
The PointerClick event is triggered when the mouse button is clicked on the Game Object.
Demonstration:
- Add an Image component to the scene. Right-click in the property window, select UI, and choose Image.
- Select the Image and click on “Add Component” in the Inspector window.
- Search for “Event Trigger” and select it from the options in the property window.
- Click on “Add New Event Type” in the Event Trigger component.
- Choose the PointerClick event from the dropdown menu.
- Now, the setup should resemble the provided image.
- Create a script and attach it to a game object. The setup should resemble the provided image.
- Copy the script below into the “TextInput” function.
using System.Collections; | |
using System.Collections.Generic; | |
using UnityEngine; | |
using UnityEngine.UI; | |
public class textInput : MonoBehaviour | |
{ | |
public void onPointerExitEventTrigger() | |
{ | |
print(“Pointer Exit Event Call……”); | |
} | |
} |
- Select the Image and click on the “+” icon to add an event.
- Drag the “Text Input script” from the Hierarchy window to the PointerClick event.
- Select the onPointerClickEventTrigger() function from the Event.
- Run the project. The function will be called when the mouse button is clicked on the Image.
Conclusion
By gaining a comprehensive understanding of the EventTrigger component in Unity and harnessing its capabilities, you are equipped with the knowledge to craft captivating and interactive user experiences within your Unity projects. The capacity to effectively respond to diverse user events opens up a vast realm of possibilities, enabling you to create immersive gameplay mechanics, seamless UI interactions, and captivating user interfaces. Embrace the power of EventTrigger, venture into its array of features, and unlock the boundless potential of interactive design, propelling your Unity creations to new heights.
Embark on a comprehensive, step-by-step journey through Event Triggers, an essential component in Unity. Unlock a deep understanding of the various types of Event Triggers and their practical applications. Implement this knowledge in your business projects to elevate their impact and success.